Getting Started with code | Web Components
Install
- npm
- Yarn
- pnpm
npm install @dhl-official/stencil-library
yarn add @dhl-official/stencil-library
pnpm add @dhl-official/stencil-library
Copy Files
info
This is example folder structure. You can adjust the folder structure according to your project. Make sure to adjust the paths in the code snippets accordingly.
Copy the following files and folder as described below to your project folder:
Name | Source package | Target folder |
---|---|---|
CSS | \node_modules\@dhl-official\tokens\dist\*.css | \css\duil\ |
Fonts | \node_modules\@dhl-official\tokens\dist\fonts\*.* | \css\duil\semantics\@dhl-official\tokens\fonts\ |
Icons | \node_modules\@dhl-official\icons\dist\*.svg | \assets\duil\icons\ |
ES Modules | \node_modules\@dhl-official\stencil-library\dist\esm\*.* | \js\duil\esm\ |
Use the script below to copy the necessary files. Run it in your project root folder using either a Bash or PowerShell terminal.
Bash script
declare -A sourcePaths=(["CSS"]="./node_modules/@dhl-official/tokens/dist/css/*" ["Fonts"]="./node_modules/@dhl-official/tokens/dist/fonts/*.*" ["Icons"]="./node_modules/@dhl-official/icons/dist/*.svg" ["ESModules"]="./node_modules/@dhl-official/stencil-library/dist/esm/*.*"); declare -A targetPaths=(["CSS"]="./css/duil/" ["Fonts"]="./css/duil/semantics/@dhl-official/tokens/fonts/" ["Icons"]="./assets/duil/icons/" ["ESModules"]="./js/duil/esm/"); copy_files() { local source=$1; local target=$2; mkdir -p "$target"; cp -r $source "$target"; }; for key in "${!sourcePaths[@]}"; do source=${sourcePaths[$key]}; target=${targetPaths[$key]}; echo "Copying $key files from $source to $target"; copy_files "$source" "$target"; done; echo "File copy operation completed."
Powershell script
$sourcePaths = @{"CSS"=".\\node_modules\\@dhl-official\\tokens\\dist\\css\\*";"Fonts"=".\\node_modules\\@dhl-official\\tokens\\dist\\fonts\\*.*";"Icons"=".\\node_modules\\@dhl-official\\icons\\dist\\*.svg";"ESModules"=".\\node_modules\\@dhl-official\\stencil-library\\dist\\esm\\*.*"}; $targetPaths = @{"CSS"=".\\css\\duil\\";"Fonts"=".\\css\\duil\\semantics\\@dhl-official\\tokens\\fonts\\";"Icons"=".\\assets\\duil\\icons\\";"ESModules"=".\\js\\duil\\esm\\"}; function Copy-Files { param ([string]$source, [string]$target) if (-not (Test-Path -Path $target)) { New-Item -ItemType Directory -Path $target -Force }; Copy-Item -Path $source -Destination $target -Recurse -Force }; foreach ($key in $sourcePaths.Keys) { $source = $sourcePaths[$key]; $target = $targetPaths[$key]; Write-Host "Copying $key files from $source to $target"; Copy-Files -source $source -target $target }; Write-Host "File copy operation completed."
After copying the files, your project folder should look like this:
project-root
│
├── css
│ └── duil
│ └── index.css
│ └── base.css
│ └── semantics.css
| └── primitives
| └── semantics
| └── @dhl-official
| └── tokens
| └── fonts
│
├── assets
│ └── duil
│ └── icons
│ └── *.svg
│
├── js
│ └── duil
│ └── esm
│ └── loader.js
│
└── index.html
Enable Custom Elements
To enable the DHL User Interface Library components in your application, you need to include the following highlighted lines in your index.html
file:
<html>
<head>
<title> DHL User Interface Library</title>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="/css/duil/index.css">
<script type="module">
import { defineCustomElements } from '/js/duil/esm/loader.js';
defineCustomElements();
var navBar = document.querySelector('dhl-navbar');
navBar.logo = {
"href": "#",
"title": "Company Name - Tagline",
"external": false,
"alt": "Company Logo"
};
</script>
<style>
body {
margin:0
}
</style>
</head>
<body>
<dhl-navbar></dhl-navbar>
<dhl-headline color="red">Welcome</dhl-headline>
<div style="width: 400px; margin: 4rem auto;">
<dhl-card>
<h1 slot="header">DHL Card Heading</h1>
<dhl-text>
Lorem ipsum, dolor sit amet consectetur adipisicing elit. Facilis a, commodi at amet sequi reprehenderit, delectus inventore vel eligendi, quibusdam numquam eaque nihil. Eligendi magni exercitationem iusto autem voluptate! Culpa?
</dhl-text>
<dhl-button slot="footer" icon="/assets/duil/icons/youtube.svg">
Click me
</dhl-button>
</dhl-card>
</div>
<dhl-footer copyright="© DHL International GmbH. All rights reserved"></dhl-footer>
</body>
</html>
Expected output
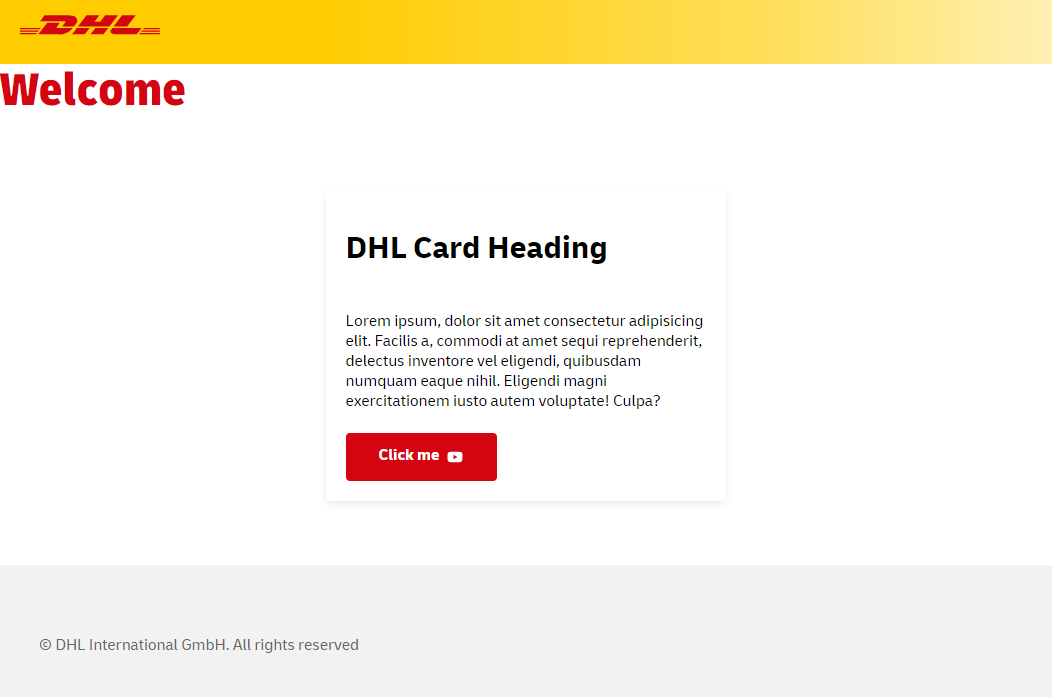
Usage
You should now be able to use DUIL components as following within your application:
<dhl-button>Button</dhl-button>